Well some of these seem less known (which I am interested in). I only know python and zsh. I’ve heard of bum. Can you tell us more, and why you like them?
All of these languages are relatively succinct, and I rely on that to reduce visual and mental clutter, because I have a pea brain.
Factor, Nim, Roc, and Zsh each offer, to differing extents, some argument-then-function ordering in the syntax, which strikes me as elegant and fun, and maybe even wise. In that order, Factor does this the most (using postfix/reverse-polish-notation and managing a data stack), and Zsh the least (piping output from one command as the input for the next command).
Roc
Roc is a functional language, and an offshoot of Elm in spirit. The lead developer and community are great. Relative to Elm, it’s more inclusive and experimental in the development process, and does not primarily or exclusively target web stuff. They aim to create an ambitiously integrated development environment, especially taking advantage of any guarantees the functional design can offer.
Here’s a sample, using the |> operator a lot, which lets you order the first argument to a function before the function IIRC:
Nim is so darn flexible and concise, has great compilation targets, and employs Uniform Function Call Syntax to more implicitly enable the kind of ordering in the Roc example. And you can often leave out parentheses entirely.
Factor
Factor is a full-on postfix and concatenative language, tons of fun, and turns my brain inside out a bit. I made a community for concatenative languages here on programming.dev, and while there’s little activity so far, I’ve filled the sidebar with a bunch of great resources, including links to active chats.
The Factor REPL (“listener”) provides excellent and speedy documentation and definitions, and a step-through debugger.
One idea that seems absurd at first is that for the most part, you don’t name data variables (though you can, and you do name function parameters). It’s all about whatever’s on the top of the stack.
In some languages it’s awkward to approximate multiple return values, but in a stack-oriented language it’s natural.
In Factor, everything is space-separated, so functions (“words”) can and do include or consist of symbols. [1..b] is not semantically something between brackets, it’s just a function that happens to be named [1..b]. It pops 1 item off the top of the stack (an integer), and pushes a range from 1 to that integer on to the top of the stack.
Here it is in my solution to the code.golf flavor of Fizz Buzz:
And in image form for glorious syntax highlighting:
Factor example walkthrough
Anything between spaced brackets is a “quotation” (lambda/anonymous function).
So:
Push a range from 1-100 onto the stack.
Push a big quotation that doesn’t end till each at the bottom.
each consumes the range and the quotation.
For each element of the range, it pushes the element then calls the quotation.
dup pushes a copy of the stack’s top item.
Say we’re in the ninth iteration of the each loop, we’ve got 9 9 on the stack.
Two quotations are pushed (99[...][...]),
then bi applies them each in turn to the single stack item directly beneath,
leaving us with 9 t f (true, it’s divisble by three, false, it’s not by 5).
2dup copies the top two, so: 9 t f t f
or combines the last two booleans: 9 t f t
Two quotes are pushed, followed by an if (9 t f t [...][...] if),
which pops that final t and calls only the first quotation.
[ drop ]2dip takes us from 9 t f to t f –
it dips under the top two, drops the temporary new top, then restores the original top two.
? is like a ternary.
That first quotation will push "Fizz" if called with t (true) on the stack, "" otherwise.
bi* applies the last two items (quotations) to the two values before them,
each only taking one value.
The Fizz one applies to t and the Buzz to f,
taking us from t f [...][...] to "Fizz"""
append joins those strings, as it would any sequence: "Fizz"
Finally we print the string, leaving us with an empty stack,
ready for the next iteration.
Hi there! Looks like you linked to a Lemmy community using a URL instead of its name, which doesn’t work well for people on different instances. Try fixing it like this: !concatenative@programming.dev
The ones I can get things done with:
My current obsession:
Honorable mentions:
Well some of these seem less known (which I am interested in). I only know python and zsh. I’ve heard of bum. Can you tell us more, and why you like them?
All of these languages are relatively succinct, and I rely on that to reduce visual and mental clutter, because I have a pea brain.
Factor, Nim, Roc, and Zsh each offer, to differing extents, some argument-then-function ordering in the syntax, which strikes me as elegant and fun, and maybe even wise. In that order, Factor does this the most (using postfix/reverse-polish-notation and managing a data stack), and Zsh the least (piping output from one command as the input for the next command).
Roc
Roc is a functional language, and an offshoot of Elm in spirit. The lead developer and community are great. Relative to Elm, it’s more inclusive and experimental in the development process, and does not primarily or exclusively target web stuff. They aim to create an ambitiously integrated development environment, especially taking advantage of any guarantees the functional design can offer.
Here’s a sample, using the
|>
operator a lot, which lets you order the first argument to a function before the function IIRC:getData = \filepath -> filepath |> Path.fromStr |> File.readUtf8 |> Task.attempt \result -> result |> Result.withDefault "" |> Task.succeed
Nim
Nim is so darn flexible and concise, has great compilation targets, and employs Uniform Function Call Syntax to more implicitly enable the kind of ordering in the Roc example. And you can often leave out parentheses entirely.
Factor
Factor is a full-on postfix and concatenative language, tons of fun, and turns my brain inside out a bit. I made a community for concatenative languages here on programming.dev, and while there’s little activity so far, I’ve filled the sidebar with a bunch of great resources, including links to active chats.
EDIT: !concatenative@programming.dev
The Factor REPL (“listener”) provides excellent and speedy documentation and definitions, and a step-through debugger.
One idea that seems absurd at first is that for the most part, you don’t name data variables (though you can, and you do name function parameters). It’s all about whatever’s on the top of the stack.
In some languages it’s awkward to approximate multiple return values, but in a stack-oriented language it’s natural.
In Factor, everything is space-separated, so functions (“words”) can and do include or consist of symbols.
[1..b]
is not semantically something between brackets, it’s just a function that happens to be named[1..b]
. It pops 1 item off the top of the stack (an integer), and pushes a range from 1 to that integer on to the top of the stack.Here it is in my solution to the code.golf flavor of Fizz Buzz:
USING: io kernel math.functions math.parser ranges sequences ; 100 [1..b] [ dup [ 3 divisor? ] [ 5 divisor? ] bi 2dup or [ [ drop ] 2dip [ "Fizz" "" ? ] [ "Buzz" "" ? ] bi* append ] [ 2drop number>string ] if print ] each
And in image form for glorious syntax highlighting: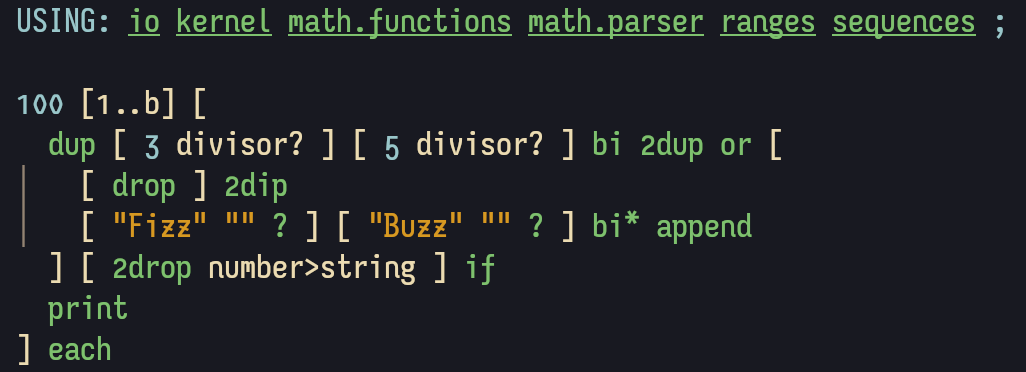
Factor example walkthrough
Anything between spaced brackets is a “quotation” (lambda/anonymous function).
So:
each
at the bottom.each
consumes the range and the quotation. For each element of the range, it pushes the element then calls the quotation.dup
pushes a copy of the stack’s top item. Say we’re in the ninth iteration of theeach
loop, we’ve got9 9
on the stack.9 9 [...] [...]
), thenbi
applies them each in turn to the single stack item directly beneath, leaving us with9 t f
(true, it’s divisble by three, false, it’s not by 5).2dup
copies the top two, so:9 t f t f
or
combines the last two booleans:9 t f t
if
(9 t f t [...] [...] if
), which pops that finalt
and calls only the first quotation.[ drop ] 2dip
takes us from9 t f
tot f
– it dips under the top two, drops the temporary new top, then restores the original top two.?
is like a ternary. That first quotation will push"Fizz"
if called witht
(true) on the stack,""
otherwise.bi*
applies the last two items (quotations) to the two values before them, each only taking one value. The Fizz one applies tot
and the Buzz tof
, taking us fromt f [...] [...]
to"Fizz" ""
append
joins those strings, as it would any sequence:"Fizz"
print
the string, leaving us with an empty stack, ready for the next iteration.EDIT: Walkthrough in image form, more granular:
Hi there! Looks like you linked to a Lemmy community using a URL instead of its name, which doesn’t work well for people on different instances. Try fixing it like this: !concatenative@programming.dev
Ah I love Factor! So many cool aspects of that language.